Firstly, let’s get a brief overview of software testing. It is an integral part of the development process, ensuring that applications perform as expected and minimizing the chances of defects. It encompasses a variety of methodologies, each designed to uncover different types of errors under various conditions.
Testers often don't have the time to check every possible input and output. While automation can handle some repetitive tests, Boundary Value Analysis (BVA) saves even more time by focusing on the edges of input values. This method helps ensure the software performs reliably by testing where the system's behavior is likely to change.
Importance of boundary value analysis (BVA)
BVA is a systematic testing method that targets the edges of input domains, where experience shows that most errors tend to occur. It is a cost-effective and time-efficient means of identifying bugs that might otherwise go unnoticed until later stages or after deployment.
In this blog, we will delve into the shades of BVA, its methodology, advantages, common challenges, and the variety of techniques that can facilitate its implementation.
The basics of Boundary Value Analysis
Definition of BVA
First, let’s answer the following question: What is BVA in software testing? BVA is a type of black-box testing technique that focuses on the values at the edge of equivalence classes. It is predicated on the principle that errors are most prevalent at the extreme ends of input ranges.
Why is BVA crucial in testing?
BVA is essential because it systematically examines boundary conditions, which are often the source of system failures and, at times, cause big troubles for the business. It helps testers efficiently allocate their efforts where they are most likely to achieve significant results. Also, the BVA technique can save a lot of time and resources used for testing.
Examples of boundaries in software applications
There are multiple boundaries in software applications, such as:
- Numeric input fields: Minimum and maximum values (e.g., age range, quantity selection).
- Date pickers: Start and end dates for a calendar selection.
- Dropdown menus: Testing the first and last options in the list.
{{cta-image}}
How Boundary Value Analysis Works
We will divide the “How Boundary Value Analysis Works” section into 2 parts, first, we will show the step-by-step process for implementation of BVA and then we’ll look at the role of fusing equivalence partitioning in BVA.
Step-by-step process for implementing BVA
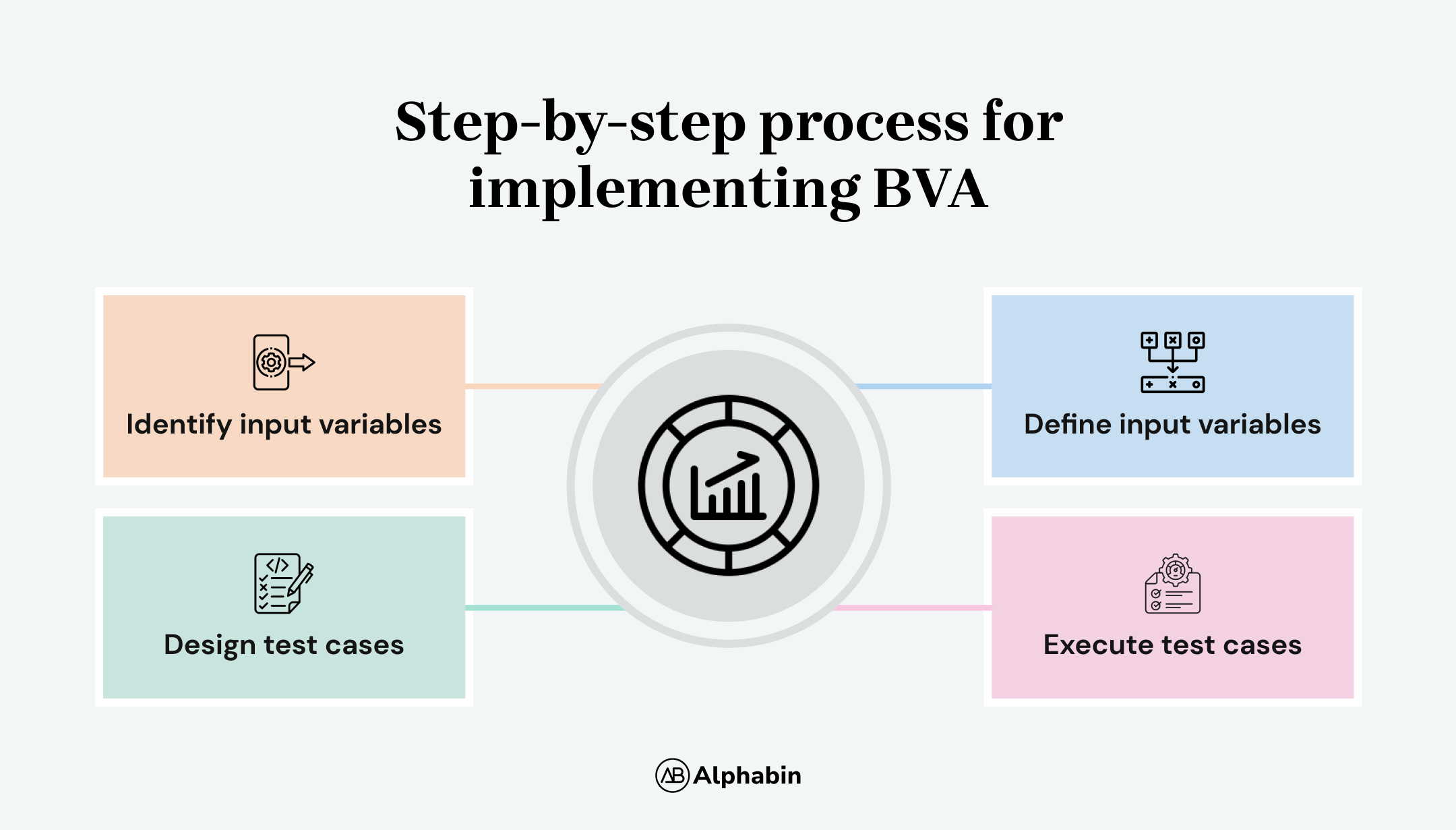
- Identify input variables
List all software functionalities that require user input. - Define input variables
Determine the valid and invalid ranges for each input variable. - Design test cases
Create test cases that target the following boundary values:- Valid boundaries (minimum, maximum)
- Values just inside the valid boundaries
- Values just outside the valid boundaries (minimum -1 and maximum + 1)
- Invalid values (e.g., negative numbers where only positives are allowed)
- Execute test cases
Run the designed test cases and analyze the software’s behavior.
The role of equivalence partitioning in Boundary Value Analysis
Equivalence partitioning is a complementary technique often used alongside BVA. It involves dividing the input values into classes (partitions), where all values within a partition are expected to behave similarly. BVA is then applied to test the boundaries of each equivalence partition for more comprehensive test coverage. The combination of ECP makes boundary testing effectiveness reach the maximum height of testing.
Advantages of boundary analysis testing
The BVA technique provides multiple advantages or benefits for software testing, such as improved test coverage, identifying potential errors, cost-saving, and time-effectiveness. Let's look at them in detail.

Improved test coverage
Boundary value analysis optimizes test coverage by pinpointing pivotal and only needed input ranges, making sure that the testing efforts are concentrated where they matter the most.
Efficiency in identifying potential errors
BVA operates with surgical precision, validating boundaries where errors are most likely to be found. Directing attention to these crucial junctures, drastically increases the chances of identifying and rectifying defects associated with input validation.
Cost-effectiveness of BVA
Time is money, and boundary value analysis in software testing proves to be a great testing investment. Through its early intervention strategy, BVA speeds up error resolution and prevents costly fixes later on. It’s like stopping a leak before it floods the house.
Time-saver
Time is incredibly valuable in software engineering. By quickly identifying key areas to focus on, BVA helps teams work more efficiently, saving time for both testers and developers. This efficient approach means teams can use their time wisely, concentrating on improving the software instead of getting stuck fixing avoidable issues.
By understanding these advantages, it becomes clear why Boundary Value Analysis is a preferred testing technique for many software development teams.
Common mistakes and How to avoid them
Now that you have knowledge about BVA and its benefits, let’s look at various mistakes that could be made by a tester while using BVA and also look at how we can avoid these mistakes via best practices.
Typical mistakes made during BVA
- Overlooking invalid boundary values
Testers sometimes ignore the invalid boundary values, which can lead to untested paths in the application, which can later be costly to fix or, worse, be found by a user. - Failing to test boundaries for all input fields
It’s common to miss testing boundaries for some input fields, especially when the application has a large number of inputs, such as a FinTech back office platform. - Not considering user experience
Selecting boundary values without considering how the end-user will interact with the application can result in a less effective test, and the product will be made without the user’s consideration.
Best practices for avoiding these mistakes
By understanding these advantages, it becomes clear why Boundary Value Analysis is a preferred testing technique for many software development teams.
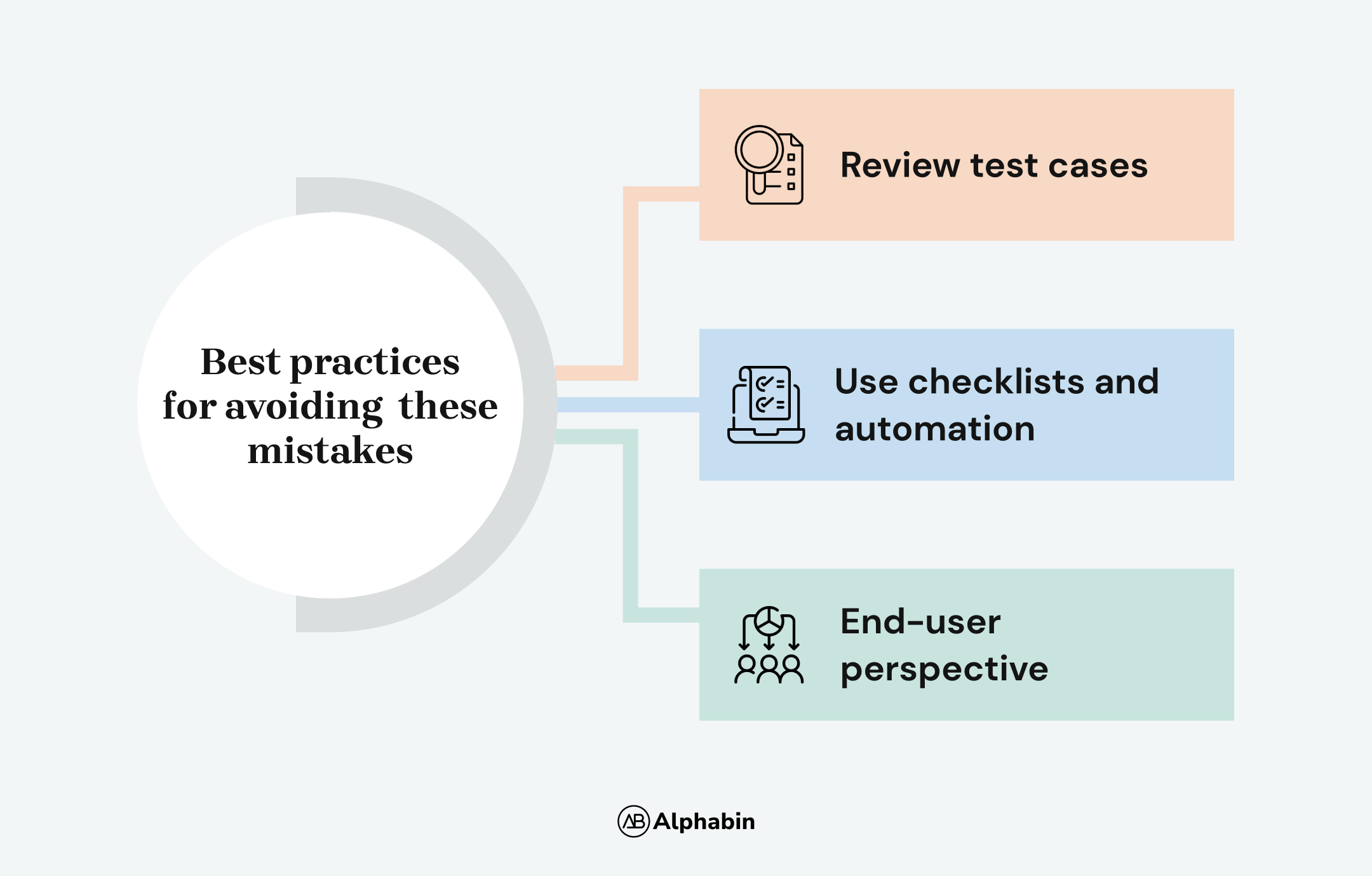
- Review test cases
- Conduct a thorough analysis of the input domain.
- Get your test cases reviewed, and make sure that the test cases cover both valid and invalid boundary values.
- Use checklists and automation
- Use checklists and automated tools to ensure no input field is overlooked.
- Develop a comprehensive test case design that includes all input fields.
- End-user perspective
- Incorporate the end-user perspective into test planning.
- Simulate real-world usage scenarios to determine practical boundary values.
Various techniques for Boundary Testing
Boundary analysis testing can be implemented with multiple techniques that will boost its effectiveness and result
Automated boundary value generation
- Utilize tools that support the automated generation of boundary values based on predefined rules.
- Integrate these tools into the testing framework to create an effective testing process.
- Run automated tests for boundary values and assess the system’s response to ensure it meets the expected outcomes.
Before heading to automation implementation, consider this manual approach.
Before automating the process, let’s understand the manual steps involved in BVA:
- Identify the input field and its valid range (e.g., “age” between 18 and 60).
- Manually input boundary values (e.g., 18, 19, 59, 60) and observe the system’s behavior.
- Verify that the application handles these values correctly.
After the manual verification, implement BVA in your automation code:
Technology used:
- Programming language: Python
- Framework: Selenium
Here’s the code implementation of automated boundary value generation:
Outcome of this BVA automation:
- Initialization
- The code initializes a Chrome WebDriver (assuming you’ve provided the correct path to the chromedriver executable).
- It sets up a 10-second wait for locating web elements.
- Boundary values
- The age_boundaries list contains the values [18, 19, 59, 60]
- Navigation
- The script navigates to the web form at “http://demo.alphabin.co”.
- Testing loop
- For each age boundary value:
- Clears the input field with ID "age".
- Enters the current age value.
- Clicks the submit button with ID "submit-button".
- Waits for the result element with ID "result" to appear.
- Prints the result text.
- For each age boundary value:
- Expected output
- The output will be printed for each age boundary value.
- The actual output depends on how the form handles these values:
- Successful submissions (e.g., “Form submitted successfully!”).
- Error messages (e.g., “Invalid age. Please enter a valid age.”).
- Any other relevant output.
Single fault assumption technique
The single fault assumption technique is a testing approach that simplifies the testing process when dealing with multiple variables. Here’s how it works:
- Hold all but one variable constant
- When testing a system with multiple variables, keep all variables fixed at their extreme values except for one.
- This means that only one variable is allowed to vary while the others remain constant.
- Test with varying variables
- Allow the remaining variables to take on their extreme values one at a time.
Execute test cases with this single variable at its minimum and maximum values.
- Allow the remaining variables to take on their extreme values one at a time.
- Why use this approach?
- By assuming that a single fault will most likely cause a failure, we reduce the number of required test cases.
- It simplifies the testing effort while still providing valuable insights into system behavior.
Let’s take a look at the code snippet for the single fault assumption technique:
Involve Equivalence Partitioning
When designing test cases, combining ECP and BVA techniques can enhance test coverage and provide thorough testing. Here’s how to do it:
- Equivalence Partitioning (ECP)
- Divide the input data into equivalent partitions based on shared characteristics.
- Each partition represents a set of values that should be treated the same.
- For example, if an input field accepts ages, we can create partitions like:
- Partition 1: Ages below 18 (Invalid)
- Partition 2: Ages between 18 and 60 (Valid)
- Partition 3: Ages above 60 (Invalid)
- Boundary Value Analysis (BVA)
- Focus on the boundary values of each partition.
- Determine representative values that encapsulate the behavior of the entire partition.
- For our age example:
- Boundary values for Partition 2 (18 to 60): Test with 18, 19, 59, and 60.
- Values just above and below the boundaries: Test with 17, 61, and other relevant values.
- Combining ECP and BVA
- Let’s combine all the above given examples and create manual test cases based on ECP and BVA.
Code snippet for involving ECP in BVA:
Let’s combine all the above given examples and create manual test cases based on ECP and BVA.
Automating the combination of BVA and ECP
Now that we have covered the manual aspect of combining ECP or involving ECP in BVA testing techniques, let’s look at how we can automate this combination.
Technology:
- Programming language: Python
- Framework: Selenium
{{cta-image-second}}
Conclusion
Quick recap: In the blog, we covered the concept of BVA, its importance in quality assurance, the methodologies for its implementation, its benefits, common mistakes while using BVA, and various techniques that will help you boost your results from using this amazing testing technique.
Final thoughts: Boundary testing plays a crucial role in enhancing your testing approach for any platform in any domain. Alphabin is the best testing services provider company in the global digital world and that helps to ensure your software is bug-free. By focusing on the most likely areas for defects to occur, this helps testers cover more tests in the limited testing time they get.